Full-Text Search with Rasa
Out of the box, Rasa Enterprise Search supports Retrieval Augmented Generation solutions with vector stores like Faiss, Milvus, and Qdrant. It also supports customizing your information retrieval,
This example extends Rasa Enterprise Search to use PostgreSQL Full Text Search.
Set up PostgreSQL database
Create a PostgreSQL database using the default user and database. Use the
password "rasa"
for this example.
Connect your database from the command line, using the PostgresSQL CLI:
The following script creates a table in the database and loads a dataset from huggingface which consists of a set of GitHub issues.
Run python ingest-data.py
to load the data into your running
PostgreSQL instance.
Create Rasa custom retrieval component
The following Python code allows Rasa to query the data loaded in the
previous step. Create directory addons
in your assistant and put this python
code in file addons/faq.py
:
Customize the EnterpriseSearchPolicy
to use the new python code:
Add the PostgreSQL credentials to endpoints.yml
:
Enable enterprise search by overriding
the default pattern_search
flow:
Train and run your assistant.
A sample chat session looks like this:
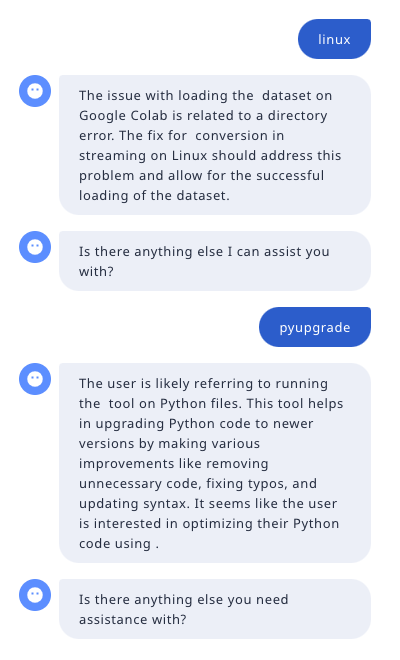